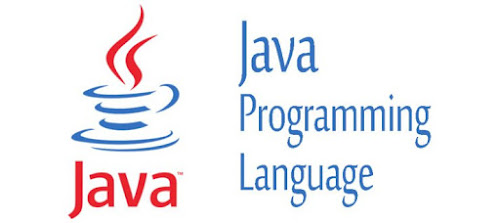
Java programming language is platform-independent language that means a Java program will run on any type of computer.
You may wonder how is this achieved? The answer lies in the fact that any Java program requires the computer it is running on to also be running a special program called a Java Virtual Machine (JVM). This JVM is able to run a Java program for the particular computer on which it is running.
For example, you can get a JVM for a PC running Windows; there is a JVM for a MacOS, and one for a Unix or Linux box. There is a special kind of JVM for mobile phones; and there are JVMs built into machines where the embedded software is written in Java.
We saw earlier that conventional compilers translate our program code into machine code. This machine code would contain the particular instructions appropriate to the type of computer it was meant for. Java compilers do not translate the program into machine code instead they translate it into special instructions call Java Byte Code. Java byte code, which, like machine code, consists of 0s and 1s, contains instructions that are exactly the same irrespective of the type of computer. It is universal, whereas machine code is specific to a particular type of computer. The job of the JVM is to translate each byte code instruction for the computer it is running on, before the instruction is performed.
There are various ways in which a JVM can be installed on a computer. In the case of some operating systems a JVM comes packaged with the system, along with the Java libraries, or packages, (pre-compiled Java modules that can be integrated with the programs you create) and a compiler. Together the JVM and the libraries are known as the Java Runtime Environment (JRE). If you do not have a JRE on your computer (as will be the case with any Windows operating system), then the entire Java Development Kit (JDK), comprising the JRE, compiler and other tools, can be downloaded from Oracle, the owners of the Java platform.
Integrated Development Environments (IDEs)
- javac MyProgram.java : This would create a file called MyProgram.class, which is the compiled file in Java byte code. The name of the JVM is java.exe and to run the program you need to type java MyProgram.
- java MyProgram : To start off with however, we strongly recommend that you use an IDE such as NetBeans or Eclipse.
Java Applications
- text based
- graphics based
0 Comments